kcl-samples → gridfinity-baseplate-magnets
gridfinity-baseplate-magnets
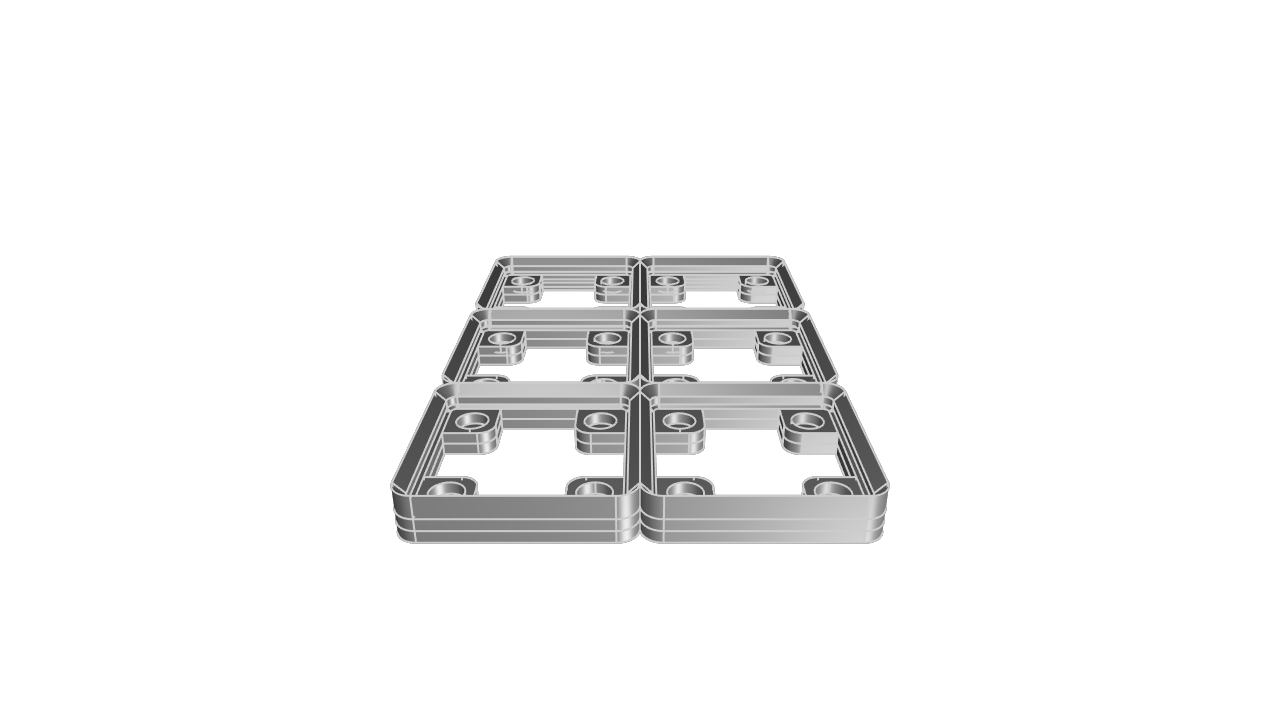
KCL
// Gridfinity Baseplate With Magnets
// Gridfinity is a system to help you work more efficiently. This is a system invented by Zack Freedman. There are two main components the baseplate and the bins. The components are comprised of a matrix of squares. Allowing easy stacking and expansion. This baseplate version includes holes for magnet placement
// Set units in millimeters (mm)
@settings(defaultLengthUnit = mm, kclVersion = 1.0)
// Define parameters
binLength = 42.0
cornerRadius = 4.0
firstStep = 0.7
secondStep = 1.8
thirdStep = 2.15
magOuterDiam = 6.5
magOffset = 4.8
magDepth = 2.4
// Number of bins in each direction
countBinWidth = 2
countBinLength = 3
// The total height of the baseplate is a summation of the vertical heights of the baseplate steps
height = firstStep + secondStep + thirdStep
// Define a function which builds the profile of the baseplate bin
fn face(@plane) {
faceSketch = startSketchOn(plane)
|> startProfile(at = [0, 0])
|> yLine(length = height)
|> angledLine(angle = -45, lengthY = thirdStep)
|> yLine(length = -secondStep)
|> angledLine(angle = -45, lengthY = firstStep)
|> close()
return faceSketch
}
// Extrude a single side of the bin
singleSide = extrude(face(offsetPlane(YZ, offset = cornerRadius)), length = binLength - (cornerRadius * 2))
// Create the other sides of the bin by using a circular pattern
sides = patternCircular3d(
singleSide,
arcDegrees = 360,
axis = [0, 0, 1],
center = [binLength / 2, binLength / 2, 0],
instances = 4,
rotateDuplicates = true,
)
// Define an axis axis000
axis000 = {
direction = [0.0, 1.0],
origin = [cornerRadius, cornerRadius]
}
// Create a single corner of the bin
singleCorner = revolve(face(offsetPlane(YZ, offset = cornerRadius)), angle = -90, axis = axis000)
// Create the corners of the bin
corners = patternCircular3d(
singleCorner,
arcDegrees = 360,
axis = [0, 0, 1],
center = [binLength / 2, binLength / 2, 0],
instances = 4,
rotateDuplicates = true,
)
// Create the baseplate by patterning sides
basePlateSides = patternLinear3d(
sides,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength)
// Create the corners of the baseplate by patterning the corners
basePlateCorners = patternLinear3d(
corners,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength)
// Create the center cutout for the magnet profile
fn magnetCenterCutout(@plane) {
magnetSketch = startSketchOn(plane)
|> startProfile(at = [
firstStep + thirdStep,
2 * magOuterDiam
])
|> xLine(length = 2 * magOuterDiam - (firstStep + thirdStep) - (magOuterDiam / 2))
|> arc(angleStart = 90.0, angleEnd = 0.0, radius = magOuterDiam / 2)
|> yLine(length = -(2 * magOuterDiam - (firstStep + thirdStep) - (magOuterDiam / 2)))
|> xLine(length = binLength - (4 * magOuterDiam))
|> yLine(length = 2 * magOuterDiam - (firstStep + thirdStep) - (magOuterDiam / 2))
|> arc(angleStart = 180.0, angleEnd = 90.0, radius = magOuterDiam / 2)
|> xLine(length = 2 * magOuterDiam - (firstStep + thirdStep) - (magOuterDiam / 2))
|> yLine(length = binLength - (4 * magOuterDiam))
|> xLine(length = -(2 * magOuterDiam - (firstStep + thirdStep) - (magOuterDiam / 2)))
|> arc(angleStart = 270.0, angleEnd = 180.0, radius = magOuterDiam / 2)
|> yLine(length = 2 * magOuterDiam - (firstStep + thirdStep) - (magOuterDiam / 2))
|> xLine(length = -(binLength - (4 * magOuterDiam)), tag = $line012)
|> yLine(length = -(2 * magOuterDiam - (firstStep + thirdStep) - (magOuterDiam / 2)))
|> arc(angleStart = 360.0, angleEnd = 270.0, radius = magOuterDiam / 2)
|> xLine(length = -(2 * magOuterDiam - (firstStep + thirdStep) - (magOuterDiam / 2)))
|> yLine(length = -(binLength - (4 * magOuterDiam)))
|> close()
return magnetSketch
}
// Create the outside profile of the magnets
fn magnetBase(@plane) {
magnetBaseSketch = startSketchOn(plane)
|> startProfile(at = [0, 0])
|> xLine(length = binLength, tag = $line001)
|> yLine(length = binLength, tag = $line002)
|> xLine(endAbsolute = profileStartX(%), tag = $line003)
|> close(tag = $line004)
|> subtract2d(tool = magnetCenterCutout(plane))
return magnetBaseSketch
}
// Create sketch profile sketch000Profile002
magnetsSketch = startSketchOn(XY)
|> circle(center = [cornerRadius * 2, cornerRadius * 2], radius = magOuterDiam / 2)
|> patternCircular2d(
center = [binLength / 2, binLength / 2],
instances = 4,
arcDegrees = 360,
rotateDuplicates = true,
)
// Create a profile with holes for the magnets
magnetProfile = magnetBase(XY)
|> subtract2d(tool = magnetsSketch)
// Create an extrusion of the magnet cutout with holes
magnetHolesExtrude = extrude(magnetProfile, length = -magDepth)
// Add a fillet to the extrusion
magnetHolesExtrudeFillets = fillet(
magnetHolesExtrude,
radius = cornerRadius,
tags = [
getNextAdjacentEdge(magnetHolesExtrude.sketch.tags.line001),
getPreviousAdjacentEdge(magnetHolesExtrude.sketch.tags.line001),
getNextAdjacentEdge(magnetHolesExtrude.sketch.tags.line003),
getPreviousAdjacentEdge(magnetHolesExtrude.sketch.tags.line003)
],
)
// Create a profile without the holes for the magnets
magnetProfileNoMagnets = magnetBase(offsetPlane(XY, offset = -magDepth))
// Create an extrusion of the magnet cutout without holes
magnetCutoutExtrude = extrude(magnetProfileNoMagnets, length = -magDepth)
// Add a fillet to the extrusion
magnetCutoutExtrudeFillets = fillet(
magnetCutoutExtrude,
radius = cornerRadius,
tags = [
getNextAdjacentEdge(magnetCutoutExtrude.sketch.tags.line001),
getPreviousAdjacentEdge(magnetCutoutExtrude.sketch.tags.line001),
getNextAdjacentEdge(magnetCutoutExtrude.sketch.tags.line003),
getPreviousAdjacentEdge(magnetCutoutExtrude.sketch.tags.line003)
],
)
// Pattern the magnet cutouts with holes
patternLinear3d(
magnetHolesExtrudeFillets,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength)
// Pattern the magnet cutouts without holes
patternLinear3d(
magnetCutoutExtrudeFillets,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength)