kcl-samples → gridfinity-bins-stacking-lip
gridfinity-bins-stacking-lip
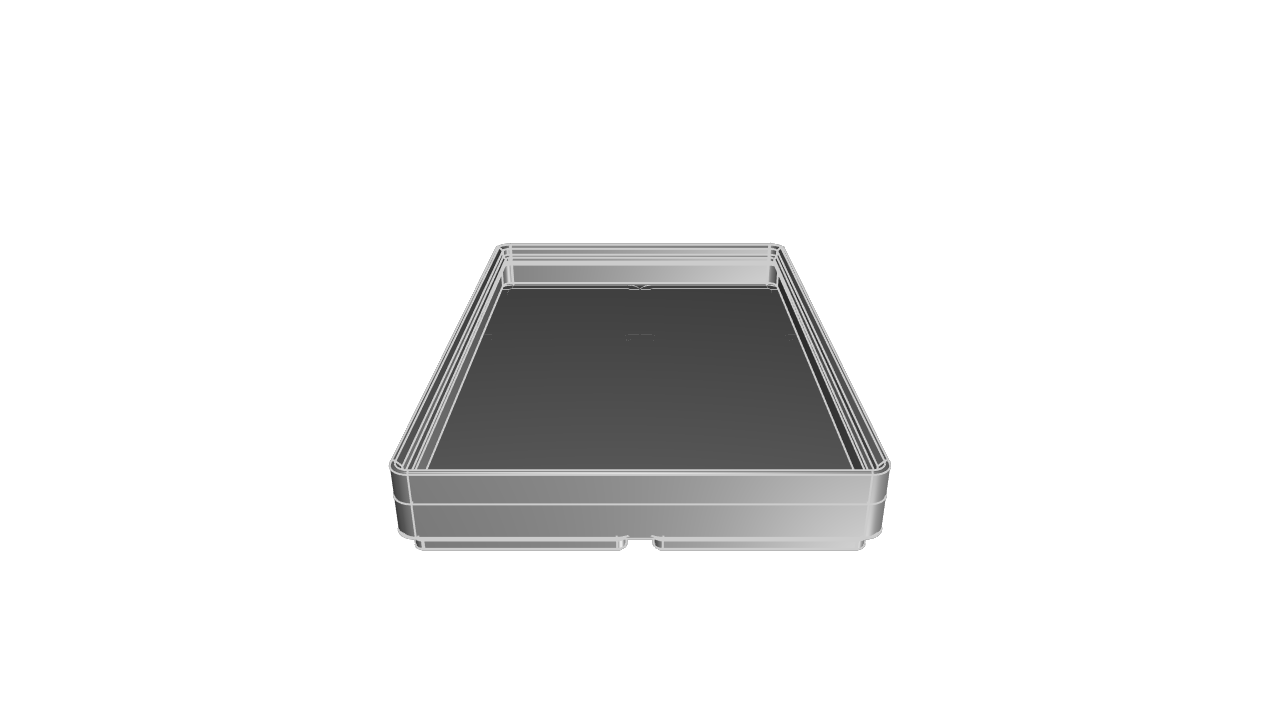
KCL
// Gridfinity Bins With A Stacking Lip
// Gridfinity is a system to help you work more efficiently. This is a system invented by Zack Freedman. There are two main components the baseplate and the bins. The components are comprised of a matrix of squares. Allowing easy stacking and expansion. This Gridfinity bins version includes a lip to allowable stacking Gridfinity bins
// Set units in millimeters (mm)
@settings(defaultLengthUnit = mm, kclVersion = 1.0)
// Define parameters
binLength = 41.5
binHeight = 7.0
binBaseLength = 2.95
binTol = 0.25
binThk = 1.2
cornerRadius = 3.75
firstStep = 0.8
secondStep = 1.8
thirdStep = 2.15
magOuterDiam = 6.5
magOffset = 4.8
magDepth = 2.4
lipRadius = 0.5
lipStep1 = 1.4
lipStep2 = 1.2
lipStep3 = 0.7
lipStep4 = 1.8
lipStep5 = 1.9
// Number of bins in each direction
countBinWidth = 2
countBinLength = 3
countBinHeight = 1
// The total height of the baseplate is a summation of the vertical heights of the baseplate steps
height = firstStep + secondStep + thirdStep
lipHeight = lipStep1 + lipStep2 + lipStep3 + lipStep4 + lipStep5
// Define a function which builds the profile of the baseplate bin
fn face(@plane) {
faceSketch = startSketchOn(plane)
|> startProfile(at = [binBaseLength + binTol, 0])
|> yLine(length = height)
|> xLine(length = -binBaseLength)
|> angledLine(angle = -45, lengthY = thirdStep)
|> yLine(length = -secondStep)
|> angledLine(angle = -45, lengthY = firstStep)
|> close()
return faceSketch
}
// Extrude a single side of the bin
singleSide = extrude(face(offsetPlane(YZ, offset = cornerRadius + binTol)), length = binLength - (cornerRadius * 2))
// Create the other sides of the bin by using a circular pattern
sides = patternCircular3d(
singleSide,
arcDegrees = 360,
axis = [0, 0, 1],
center = [
(binLength + 2 * binTol) / 2,
(binLength + 2 * binTol) / 2,
0
],
instances = 4,
rotateDuplicates = true,
)
// Define an axis axis000
axis000 = {
direction = [0.0, 1.0],
origin = [
cornerRadius + binTol,
cornerRadius + binTol
]
}
// Create a single corner of the bin
singleCorner = revolve(face(offsetPlane(YZ, offset = cornerRadius + binTol)), angle = -90, axis = axis000)
// Create the corners of the bin
corners = patternCircular3d(
singleCorner,
arcDegrees = 360,
axis = [0, 0, 1],
center = [
(binLength + 2 * binTol) / 2,
(binLength + 2 * binTol) / 2,
0
],
instances = 4,
rotateDuplicates = true,
)
singleBinFill = startSketchOn(XY)
|> startProfile(at = [
binBaseLength + binTol,
binBaseLength + binTol
])
|> line(end = [binLength - (binBaseLength * 2), 0], tag = $line000)
|> line(end = [0, binLength - (binBaseLength * 2)], tag = $line001)
|> xLine(endAbsolute = profileStartX(%), tag = $line002)
|> close(tag = $line003)
|> extrude(length = height)
|> fillet(
radius = firstStep,
tags = [
getNextAdjacentEdge(line000),
getPreviousAdjacentEdge(line000),
getNextAdjacentEdge(line002),
getPreviousAdjacentEdge(line002)
],
)
magCutout000 = startSketchOn(singleBinFill, face = START)
|> circle(
center = [
-magOffset - binBaseLength - binTol,
magOffset + binBaseLength + binTol
],
radius = magOuterDiam / 2,
)
|> patternCircular2d(
arcDegrees = 360,
center = [
(-binLength + 2 * binTol) / 2,
(binLength + 2 * binTol) / 2
],
instances = 4,
rotateDuplicates = true,
)
|> extrude(length = -magDepth)
// Create the baseplate by patterning sides
binSides = patternLinear3d(
sides,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength + binTol * 2,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength + binTol * 2)
// Create the corners of the baseplate by patterning the corners
binCorners = patternLinear3d(
corners,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength + binTol * 2,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength + binTol * 2)
// Create the fill of the bin by patterning the corners
binFill = patternLinear3d(
singleBinFill,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength + binTol * 2,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength + binTol * 2)
binTop = startSketchOn(offsetPlane(XY, offset = height))
|> startProfile(at = [0, 0])
|> xLine(length = (binLength + 2 * binTol) * countBinWidth, tag = $line010)
|> yLine(length = (binLength + 2 * binTol) * countBinLength, tag = $line011)
|> xLine(endAbsolute = profileStartX(%), tag = $line012)
|> close(tag = $line013)
|> extrude(length = binHeight * countBinHeight)
|> fillet(
radius = cornerRadius,
tags = [
getNextAdjacentEdge(line010),
getPreviousAdjacentEdge(line010),
getNextAdjacentEdge(line012),
getPreviousAdjacentEdge(line012)
],
)
|> shell(faces = [END], thickness = binThk)
// Define a function which builds the profile of the baseplate bin
fn lipFace(@plane) {
faceSketch = startSketchOn(plane)
|> startProfile(at = [0, 0])
// |> yLine(length = lipHeight, tag = $line100)
|> line(end = [0.0, 5.792893], tag = $line000)
|> arc(
angleStart = 180.0,
angleEnd = 45.0,
radius = 0.500000,
tag = $arc000,
)
// |> angledLine(angle = -45, lengthY = lipStep5 )
|> line(end = [1.046447, -1.046447], tag = $line001)
|> yLine(length = -lipStep4)
|> angledLine(angle = -45, lengthY = lipStep3)
|> yLine(length = -lipStep2)
|> angledLine(angle = -135, lengthY = lipStep1)
|> close()
return faceSketch
}
plane000 = {
origin = [
cornerRadius,
0.0,
height + binHeight * countBinHeight
],
xAxis = [0.0, 1.0, 0.0],
yAxis = [0.0, 0.0, 1.0]
}
plane001 = {
origin = [
0.0,
countBinLength * (binLength + 2 * binTol) - cornerRadius,
height + binHeight * countBinHeight
],
xAxis = [1.0, 0.0, 0.0],
yAxis = [0.0, 0.0, 1.0]
}
plane002 = {
origin = [
countBinWidth * (binLength + 2 * binTol) - cornerRadius,
0.0,
height + binHeight * countBinHeight
],
xAxis = [0.0, 1.0, 0.0],
yAxis = [0.0, 0.0, 1.0]
}
// Extrude a single side of the lip of the bin
lipSingleLength = extrude(lipFace(plane000), length = binLength * countBinWidth - (2 * cornerRadius) + 2 * binTol * countBinWidth)
// Extrude a single side of the lip of the bin
lipSingleWidth = extrude(lipFace(plane001), length = binLength * countBinLength - (2 * cornerRadius) + 2 * binTol * countBinLength)
// Create the other sides of the lips by using a circular pattern
lipLengths = patternCircular3d(
lipSingleLength,
arcDegrees = 360,
axis = [0, 0, 1],
center = [
(binLength + 2 * binTol) / 2 * countBinWidth,
(binLength + 2 * binTol) / 2 * countBinLength,
0
],
instances = 2,
rotateDuplicates = true,
)
// Create the other sides of the lips by using a circular pattern
lipWidths = patternCircular3d(
lipSingleWidth,
arcDegrees = 360,
axis = [0, 0, 1],
center = [
(binLength + 2 * binTol) / 2 * countBinWidth,
(binLength + 2 * binTol) / 2 * countBinLength,
0
],
instances = 2,
rotateDuplicates = true,
)
// Define an axis axis000
axis001 = {
direction = [0.0, 1.0],
origin = [cornerRadius, cornerRadius]
}
// Create a single corner of the bin
lipSingleLengthCorner = revolve(lipFace(plane000), angle = -90, axis = axis001)
// Create a single corner of the bin
lipSingleWidthCorner = revolve(lipFace(plane002), angle = 90, axis = axis001)
// Create the corners of the bin
lipCorners000 = patternCircular3d(
lipSingleLengthCorner,
arcDegrees = 360,
axis = [0, 0, 1],
center = [
(binLength + 2 * binTol) / 2 * countBinWidth,
(binLength + 2 * binTol) / 2 * countBinLength,
0
],
instances = 2,
rotateDuplicates = true,
)
// Create the corners of the bin
lipCorners001 = patternCircular3d(
lipSingleWidthCorner,
arcDegrees = 360,
axis = [0, 0, 1],
center = [
(binLength + 2 * binTol) / 2 * countBinWidth,
(binLength + 2 * binTol) / 2 * countBinLength,
0
],
instances = 2,
rotateDuplicates = true,
)