kcl-samples → food-service-spatula
food-service-spatula
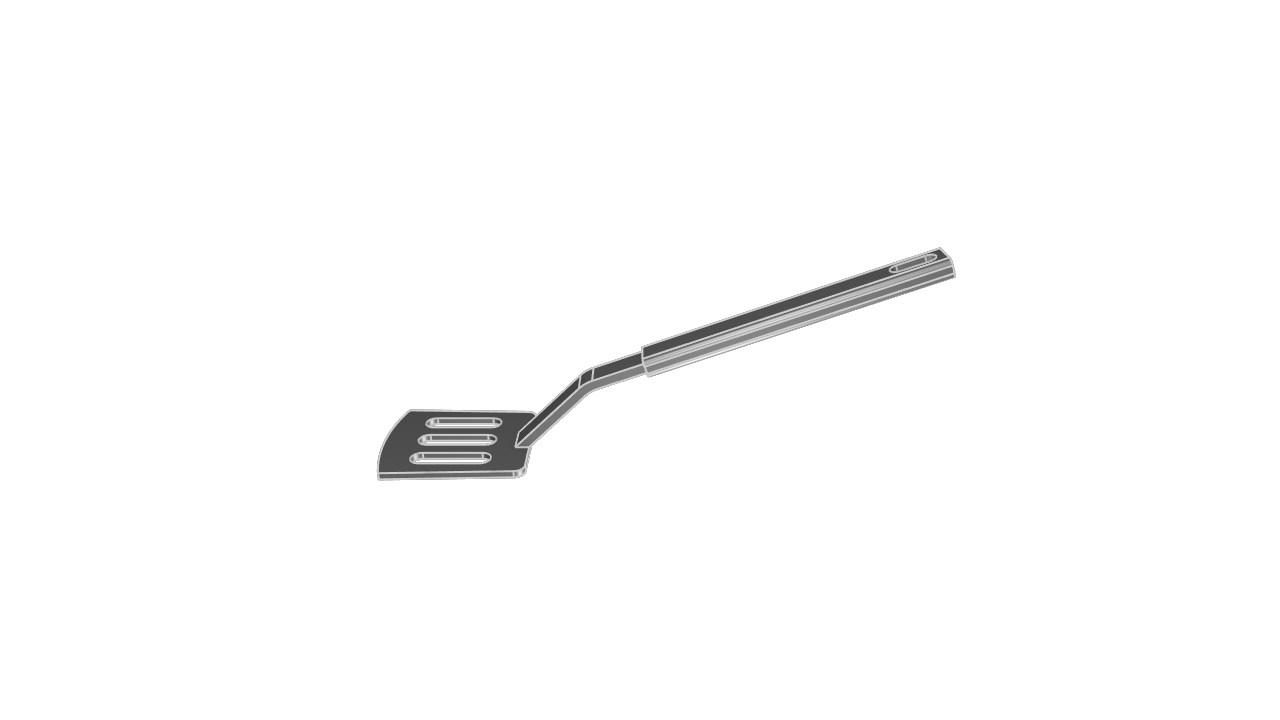
KCL
// Food Service Spatula
// Use these spatulas for mixing, flipping, and scraping.
// Set units
@settings(defaultLengthUnit = mm, kclVersion = 1.0)
// Define parameters
flipperThickness = 3.5
flipperLength = 70.0
handleWidth = 15.0
gripLength = 150.0
flipperFilletRadius = 5.0
flipperSlotWidth = 10.0
gripWidth = 10.0
gripHeight = 20.0
gripFilletRadius = 3.0
gripSlotWidth = 8.0
// Function for drawing slots on a sketch given the start and end points as well as a width
fn slot(sketch1, start, end, width) {
angle = if start[0] == end[0] {
if end[1] > start[1] {
90
} else {
270
}
} else {
if end[0] < start[0] {
units::toDegrees(atan((end[1] - start[1]) / (end[0] - start[0]))) + 180
} else {
units::toDegrees(atan((end[1] - start[1]) / (end[0] - start[0])))
}
}
dist = sqrt(pow(end[1] - start[1], exp = 2) + pow(end[0] - start[0], exp = 2))
xstart = width / 2 * cos(angle - 90) + start[0]
ystart = width / 2 * sin(angle - 90) + start[1]
slotSketch = startProfile(sketch1, at = [xstart, ystart])
|> angledLine(angle = angle, length = dist)
|> tangentialArc(radius = width / 2, angle = 180)
|> angledLine(angle = angle, length = -dist)
|> tangentialArc(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
return slotSketch
}
// Create a sketch on the XY plane for the flipper
flipperSketch = startSketchOn(XY)
// Create a profile of the flipper
flipperProfile = startProfile(flipperSketch, at = [-flipperLength, -32.0])
|> line(end = [flipperLength, 2.0])
|> yLine(length = 60.0, tag = $backEdge)
|> line(end = [-flipperLength, 2.0])
|> arc(angleStart = 163.087610, angleEnd = 196.912390, radius = 110.0)
|> close()
// Create a profile of the middle
slotProfile000 = slot(
sketch1 = flipperSketch,
start = [-25, 0],
end = [-55, 0],
width = flipperSlotWidth,
)
// Create a profile of the top slot
slotProfile001 = slot(
sketch1 = flipperSketch,
start = [-25, 18],
end = [-55, 19],
width = flipperSlotWidth,
)
// Create a profile of the bottom slot
slotProfile002 = slot(
sketch1 = flipperSketch,
start = [-25, -18],
end = [-55, -19],
width = flipperSlotWidth,
)
// Create a profile with slots for the spatula
spatulaProfile = flipperProfile
|> subtract2d(tool = slotProfile000)
|> subtract2d(tool = slotProfile001)
|> subtract2d(tool = slotProfile002)
// Extrude the profile to create the spatula flipper
flipper = extrude(spatulaProfile, length = flipperThickness)
// Fillet the edges of the flipper
fillet(
flipper,
radius = flipperFilletRadius,
tags = [
getNextAdjacentEdge(backEdge),
getPreviousAdjacentEdge(backEdge)
],
)
// Create a sketch on the XZ plane offset by half the thickness
handleSketch = startSketchOn(offsetPlane(XZ, offset = -handleWidth / 2))
// Create a profile of the spatula handle
handleProfile = startProfile(handleSketch, at = [0.0, flipperThickness])
|> line(end = [31.819805, 31.819805], tag = $handleBottomEdge)
|> line(end = [140.953893, 51.303021])
|> line(end = [-1.710101, 4.698463])
|> line(end = [-141.995517, -51.682142], tag = $handleTopEdge)
|> line(end = [-36.139148, -36.139148])
|> xLine(length = 7.071068)
|> close()
// Create an extrusion
handle = extrude(handleProfile, length = handleWidth)
// Fillet the bend of the spatula handle
fillet(
handle,
radius = 4,
tags = [
getNextAdjacentEdge(handleBottomEdge),
getNextAdjacentEdge(handleTopEdge)
],
)
// Define a plane which is at the end of the handle
handlePlane = {
origin = [208.593833, 0.0, 75.921946],
xAxis = [0.342020, -0.0, -0.939693],
yAxis = [0.0, 1.0, 0.0],
zAxis = [0.939693, -0.0, 0.342020]
}
// Create a sketch on the handle plane
gripSketch = startSketchOn(handlePlane)
// Create a profile of the grip
gripProfile = startProfile(gripSketch, at = [-26.806746, -10.0])
|> xLine(length = gripWidth - (2 * gripFilletRadius))
|> arc(angleStart = -90.0, angleEnd = 0.0, radius = gripFilletRadius)
|> yLine(length = gripHeight - (2 * gripFilletRadius))
|> arc(angleStart = 0.0, angleEnd = 90.0, radius = gripFilletRadius)
|> xLine(length = -(gripWidth - (2 * gripFilletRadius)))
|> arc(angleStart = 90.0, angleEnd = 180.0, radius = gripFilletRadius)
|> yLine(length = -(gripHeight - (2 * gripFilletRadius)), tag = $gripEdgeTop)
|> arc(angleStart = 180.0, angleEnd = 270.0, radius = gripFilletRadius)
|> close()
// Extrude the grip profile to create the grip
grip = extrude(gripProfile, length = -gripLength)
// Create a sketch on the grip for the hole
holeSketch = startSketchOn(grip, face = gripEdgeTop)
// Create a profile for the grip hole
gripHoleProfile = slot(
sketch1 = holeSketch,
start = [0, 200],
end = [0, 210],
width = gripSlotWidth,
)
// Cut a hole in the grip
extrude(gripHoleProfile, length = -gripWidth - 20)