kcl-samples → gridfinity-baseplate
gridfinity-baseplate
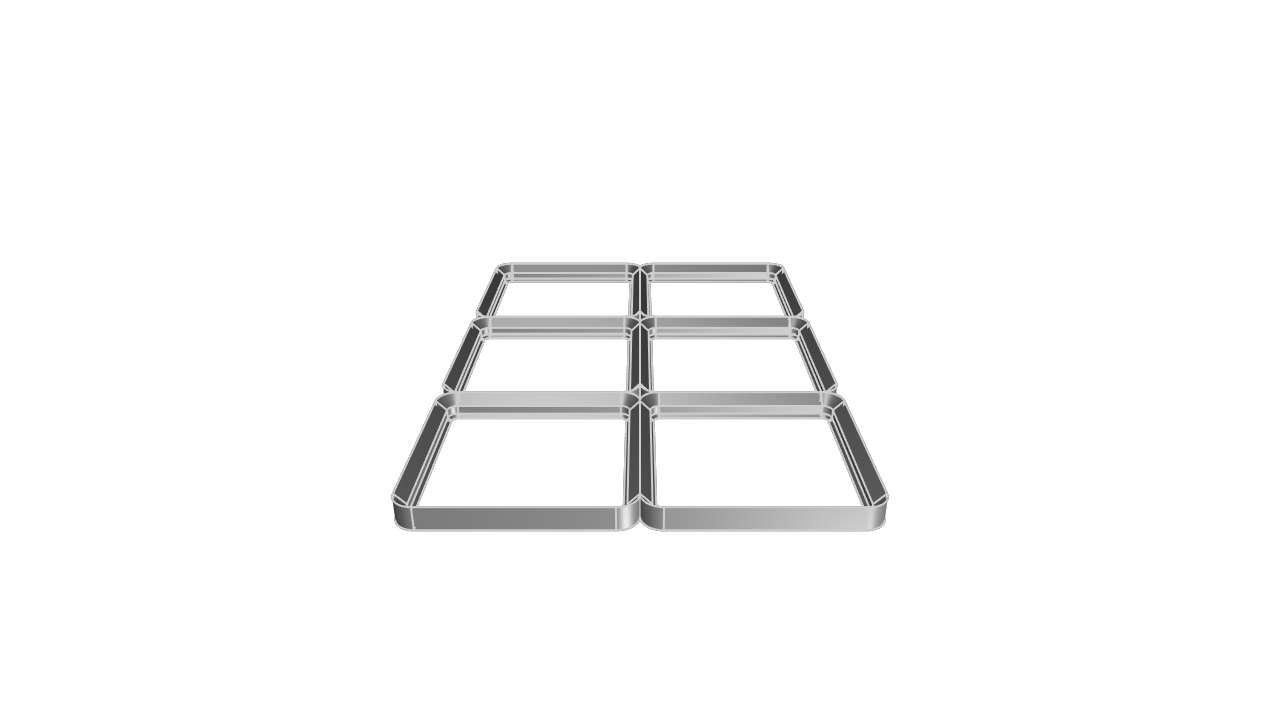
KCL
// Gridfinity Baseplate
// Gridfinity is a system to help you work more efficiently. This is a system invented by Zack Freedman. There are two main components the baseplate and the bins. The components are comprised of a matrix of squares. Allowing easy stacking and expansion
// Set units in millimeters (mm)
@settings(defaultLengthUnit = mm, kclVersion = 1.0)
// Define parameters
binLength = 42.0
cornerRadius = 4.0
firstStep = 0.7
secondStep = 1.8
thirdStep = 2.15
// Number of bins in each direction
countBinWidth = 2
countBinLength = 3
// The total height of the baseplate is a summation of the vertical heights of the baseplate steps
height = firstStep + secondStep + thirdStep
// Define a function which builds the profile of the baseplate bin
fn face(@plane) {
faceSketch = startSketchOn(plane)
|> startProfile(at = [0, 0])
|> yLine(length = height)
|> angledLine(angle = -45, lengthY = thirdStep)
|> yLine(length = -secondStep)
|> angledLine(angle = -45, lengthY = firstStep)
|> close()
return faceSketch
}
// Extrude a single side of the bin
singleSide = extrude(face(offsetPlane(YZ, offset = cornerRadius)), length = binLength - (cornerRadius * 2))
// Create the other sides of the bin by using a circular pattern
sides = patternCircular3d(
singleSide,
arcDegrees = 360,
axis = [0, 0, 1],
center = [binLength / 2, binLength / 2, 0],
instances = 4,
rotateDuplicates = true,
)
// Define an axis axis000
axis000 = {
direction = [0.0, 1.0],
origin = [cornerRadius, cornerRadius]
}
// Create a single corner of the bin
singleCorner = revolve(face(offsetPlane(YZ, offset = cornerRadius)), angle = -90, axis = axis000)
// Create the corners of the bin
corners = patternCircular3d(
singleCorner,
arcDegrees = 360,
axis = [0, 0, 1],
center = [binLength / 2, binLength / 2, 0],
instances = 4,
rotateDuplicates = true,
)
// Create the baseplate by patterning sides
basePlateSides = patternLinear3d(
sides,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength)
// Create the corners of the baseplate by patterning the corners
basePlateCorners = patternLinear3d(
corners,
axis = [1.0, 0.0, 0.0],
instances = countBinWidth,
distance = binLength,
)
|> patternLinear3d(axis = [0.0, 1.0, 0.0], instances = countBinLength, distance = binLength)