kcl-samples → multi-axis-robot →
multi-axis-robot
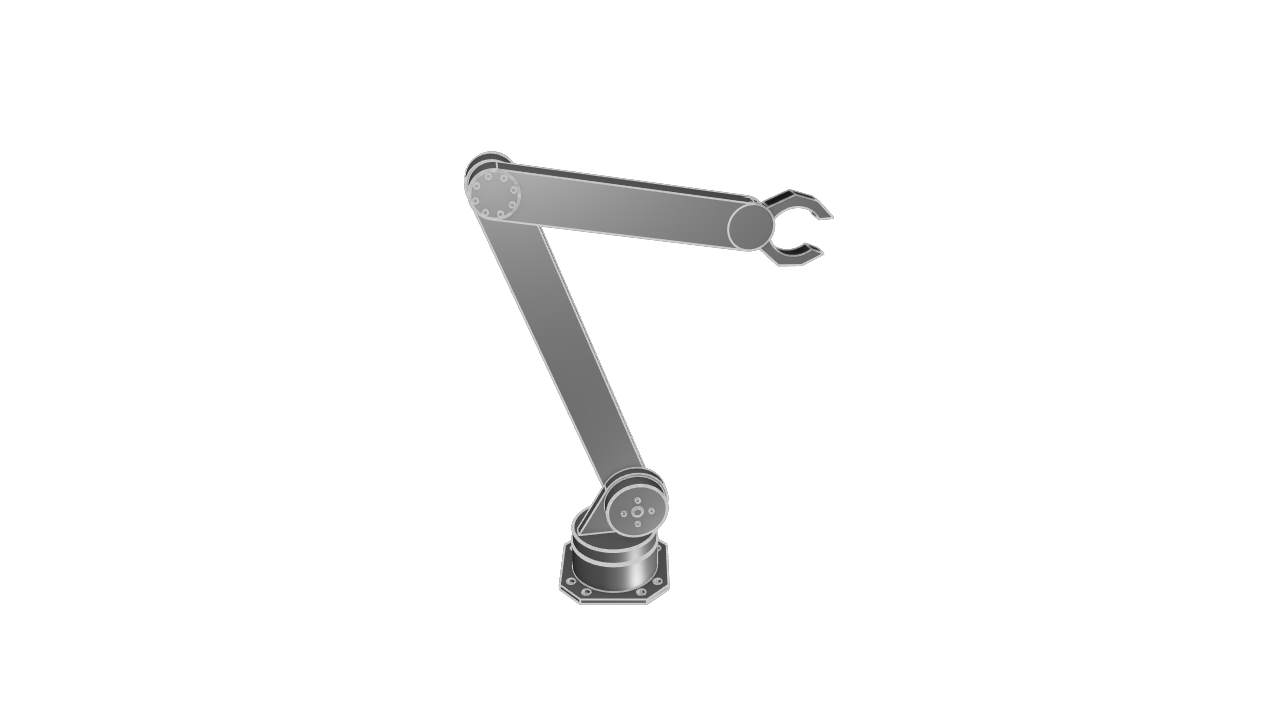
KCL
// Robot Arm
// A 4 axis robotic arm for industrial use. These machines can be used for assembly, packaging, organization of goods, and quality inspection processes
const axisJ4 = 25
const axisJ3 = 60
const axisJ2 = 110
const axisJ1 = 80
// Robot Arm Base
const basePlateRadius = 5
const basePlateThickness = 0.5
const baseChamfer = 2
const baseHeight = 5
const sketch001 = startSketchOn('XY')
|> startProfileAt([-basePlateRadius, -basePlateRadius], %)
|> angledLine([0, 2 * basePlateRadius], %, $rectangleSegmentA001)
|> angledLine([
segAng(rectangleSegmentA001) + 90,
2 * basePlateRadius
], %, $rectangleSegmentB001)
|> angledLine([
segAng(rectangleSegmentA001),
-segLen(rectangleSegmentA001)
], %, $rectangleSegmentC001)
|> lineTo([profileStartX(%), profileStartY(%)], %, $rectangleSegmentD001)
|> close(%)
const extrude001 = extrude(basePlateThickness, sketch001)
|> chamfer({
length: baseChamfer,
tags: [
getNextAdjacentEdge(rectangleSegmentA001),
getNextAdjacentEdge(rectangleSegmentB001),
getNextAdjacentEdge(rectangleSegmentC001),
getNextAdjacentEdge(rectangleSegmentD001)
]
}, %)
// Base Motor for actuating first joint
const sketch002 = startSketchOn(extrude001, 'END')
|> circle([0, 0], 4, %, $referenceEdge)
const extrude002 = extrude(baseHeight - basePlateThickness - 1.5, sketch002)
|> fillet({
radius: 0.1,
tags: [getOppositeEdge(referenceEdge)]
}, %)
const sketch003 = startSketchOn(extrude002, 'END')
|> circle([0, 0], 0.5, %)
const extrude003 = extrude(1, sketch003)
// Pattern M8 mounting bolts in base
const sketch4A = startSketchOn(extrude001, 'END')
|> circle([
-basePlateRadius + 1,
-basePlateRadius + baseChamfer + 0.5
], 0.4, %)
|> patternCircular2d({
arcDegrees: 360,
center: [0, 0],
repetitions: 3,
rotateDuplicates: true
}, %)
const extrude4A = extrude(-basePlateThickness, sketch4A)
const sketch4B = startSketchOn(extrude001, 'END')
|> circle([
-basePlateRadius + 0.5 + baseChamfer,
-basePlateRadius + 1
], 0.4, %)
|> patternCircular2d({
arcDegrees: 360,
center: [0, 0],
repetitions: 3,
rotateDuplicates: true
}, %)
const extrude4B = extrude(-basePlateThickness, sketch4B)
// Housing for J2 motor
// Define Planes to change robot angle
const plane001 = {
plane: {
origin: [0.0, 0.0, baseHeight - 1.5 + 0.1],
xAxis: [1.0, 0.0, 0.0],
yAxis: [0.0, 1.0, 0.0],
zAxis: [0.0, 0.0, 1.0]
}
}
const plane002 = {
plane: {
origin: [0.0, 0.0, 0.0],
xAxis: [
sin( toRadians(axisJ1)),
cos( toRadians(axisJ1)),
0.0
],
yAxis: [0.0, 0.0, 1.0],
zAxis: [1.0, 0.0, 0.0]
}
}
// Create Rotating Base
const sketch005 = startSketchOn(plane001)
|> circle([0, 0], 3.9, %, $referenceEdge1)
const extrude005 = extrude(1.5 - 0.1, sketch005)
|> fillet({
radius: 0.1,
tags: [getOppositeEdge(referenceEdge1)]
}, %)
const sketch006 = startSketchOn(plane002)
|> startProfileAt([3.5, baseHeight], %)
|> angledLine({ angle: 60, length: 1.75 }, %)
|> arc({
angleStart: -30,
angleEnd: -30 + 180,
radius: 3
}, %)
|> angledLineToY({ angle: 60, to: baseHeight }, %)
|> lineTo([profileStartX(%), profileStartY(%)], %)
|> close(%)
const extrude006 = extrude(1, sketch006)
const sketch007 = startSketchOn(extrude006, 'END')
|> circle([
1.75 * cos(toRadians(axisJ1)) / abs(cos(toRadians(axisJ1))),
8
], 2.75, %, $referenceEdge2)
const extrude007 = extrude(1.5, sketch007)
|> fillet({
radius: 0.1,
tags: [getOppositeEdge(referenceEdge2)]
}, %)
// Draw Bolt Pattern on Rotating Base
const sketch008 = startSketchOn(extrude007, 'END')
|> circle([
1.75 * cos(toRadians(axisJ1)) / abs(cos(toRadians(axisJ1))),
6.75
], 0.2, %)
|> patternCircular2d({
center: [
1.75 * cos(toRadians(axisJ1)) / abs(cos(toRadians(axisJ1))),
8
],
repetitions: 3,
arcDegrees: 360,
rotateDuplicates: true
}, %)
const extrude008 = extrude(0.2, sketch008)
const sketch009 = startSketchOn(extrude007, 'END')
|> circle([
1.75 * cos(toRadians(axisJ1)) / abs(cos(toRadians(axisJ1))),
8
], 0.5, %, $referenceEdge3)
const extrude009 = extrude(0.15, sketch009)
|> fillet({
radius: 0.1,
tags: [getOppositeEdge(referenceEdge3)]
}, %)
const sketch010 = startSketchOn(plane002)
|> circle([1.75, 8], 0.3, %)
const extrude010 = extrude(-1, sketch010)
// J2 Axis for Robot Arm
// Define Constants for J2 Axis Robot Arm
const axisJ2ArmLength = 31
const axisJ2ArmWidth = 4
const axisJ2ArmThickness = 2.5
// Define Plane to Move J2 Axis Robot Arm
const plane003 = {
plane: {
origin: [-0.1, 0.0, 0.0],
xAxis: [
sin( toRadians(axisJ1)),
cos( toRadians(axisJ1)),
0.0
],
yAxis: [0.0, 0.0, 1.0],
zAxis: [1.0, 0.0, 0.0]
}
}
// Create Body of J2 Robot Arm
const sketch011 = startSketchOn(plane003)
|> startProfileAt([
1.75 - (axisJ2ArmWidth / 2 * sin(toRadians(axisJ2))),
8 + axisJ2ArmWidth / 2 * cos(toRadians(axisJ2))
], %)
|> arc({
angleStart: 90 + axisJ2,
angleEnd: 270 + axisJ2,
radius: axisJ2ArmWidth / 2
}, %)
|> angledLine({
angle: axisJ2,
length: axisJ2ArmLength
}, %)
|> arc({
angleStart: -90 + axisJ2,
angleEnd: 90 + axisJ2,
radius: axisJ2ArmWidth / 2
}, %)
|> lineTo([profileStartX(%), profileStartY(%)], %)
|> close(%)
const extrude011 = extrude(-axisJ2ArmThickness, sketch011)
const sketch012 = startSketchOn(extrude011, 'START')
|> circle([-1.75, 8], 1.9, %, $referenceEdge4)
const extrude012 = extrude(0.15, sketch012)
|> fillet({
radius: 0.1,
tags: [getOppositeEdge(referenceEdge4)]
}, %)
const sketch013 = startSketchOn(extrude011, 'START')
|> circle([
-1.75 - (axisJ2ArmLength * cos(toRadians(axisJ2))),
8 + axisJ2ArmLength * sin(toRadians(axisJ2))
], 1.9, %, $referenceEdge5)
const extrude013 = extrude(1, sketch013)
|> fillet({
radius: 0.1,
tags: [getOppositeEdge(referenceEdge5)]
}, %)
// Draw Bolt Patterns on J2 Robot Arm
const sketch014 = startSketchOn(extrude012, 'END')
|> circle([-1.75, 6.75], 0.2, %)
|> patternCircular2d({
center: [-1.75, 8],
repetitions: 7,
arcDegrees: 360,
rotateDuplicates: true
}, %)
const extrude014 = extrude(0.15, sketch014)
const sketch015 = startSketchOn(extrude013, 'END')
|> circle([
-1.75 - ((axisJ2ArmLength - 1) * cos(toRadians(axisJ2))),
8 + (axisJ2ArmLength - 1.5) * sin(toRadians(axisJ2))
], 0.2, %)
|> patternCircular2d({
center: [
-1.75 - (axisJ2ArmLength * cos(toRadians(axisJ2))),
8 + axisJ2ArmLength * sin(toRadians(axisJ2))
],
repetitions: 3,
arcDegrees: 360,
rotateDuplicates: true
}, %)
const extrude015 = extrude(0.15, sketch015)
const sketch016 = startSketchOn(extrude011, 'END')
|> circle([
1.75 + axisJ2ArmLength * cos(toRadians(axisJ2)),
8 + axisJ2ArmLength * sin(toRadians(axisJ2))
], 0.3, %)
const extrude016 = extrude(1, sketch016)
// J3 Axis for Robot Arm
const axisJ3C = axisJ3 - 180 + axisJ2
const axisJ3CArmLength = 20
const axisJ3CArmWidth = 3.75
const axisJ3CArmThickness = 2.5
// Create Body of J3 Robot Arm
const sketch017 = startSketchOn(plane002)
|> startProfileAt([
1.75 + axisJ2ArmLength * cos(toRadians(axisJ2)) - (axisJ3CArmWidth / 2 * sin(toRadians(axisJ3C))),
8 + axisJ2ArmLength * sin(toRadians(axisJ2)) + axisJ3CArmWidth / 2 * cos(toRadians(axisJ3C))
], %)
|> arc({
angleStart: 90 + axisJ3C,
angleEnd: 270 + axisJ3C,
radius: axisJ3CArmWidth / 2
}, %)
|> angledLine({
angle: axisJ3C,
length: axisJ3CArmLength
}, %)
|> arc({
angleStart: 270 + axisJ3C,
angleEnd: 90 + axisJ3C,
radius: axisJ3CArmWidth / 2
}, %)
|> lineTo([profileStartX(%), profileStartY(%)], %, $seg01)
|> close(%)
const extrude017 = extrude(axisJ3CArmThickness, sketch017)
const sketch018 = startSketchOn(extrude017, 'END')
|> circle([
1.75 + axisJ2ArmLength * cos(toRadians(axisJ2)),
8 + axisJ2ArmLength * sin(toRadians(axisJ2))
], 3.7 / 2, %, $referenceEdge6)
const extrude018 = extrude(0.15, sketch018)
|> fillet({
radius: 0.1,
tags: [getOppositeEdge(referenceEdge6)]
}, %)
// Draw Bolt Pattern on J3 Robot Arm
const sketch019 = startSketchOn(extrude018, 'END')
|> circle([
1.75 + (axisJ2ArmLength - 1) * cos(toRadians(axisJ2)),
8 + (axisJ2ArmLength - 1.5) * sin(toRadians(axisJ2))
], 0.2, %)
|> patternCircular2d({
center: [
1.75 + axisJ2ArmLength * cos(toRadians(axisJ2)),
8 + axisJ2ArmLength * sin(toRadians(axisJ2))
],
repetitions: 7,
arcDegrees: 360,
rotateDuplicates: true
}, %)
const extrude019 = extrude(0.15, sketch019)
// On the J3 Robot Arm Body, Create Mounting Clevis for Grabber Claw
const sketch020 = startSketchOn(extrude017, 'START')
|> circle([
-1.75 - (axisJ2ArmLength * cos(toRadians(axisJ2))) - (axisJ3CArmLength * cos(toRadians(axisJ3C))),
8 + axisJ2ArmLength * sin(toRadians(axisJ2)) + axisJ3CArmLength * sin(toRadians(axisJ3C))
], axisJ3CArmWidth / 2, %)
const extrude020 = extrude(-0.5, sketch020)
const sketch021 = startSketchOn(extrude017, 'END')
|> circle([
1.75 + axisJ2ArmLength * cos(toRadians(axisJ2)) + axisJ3CArmLength * cos(toRadians(axisJ3C)),
8 + axisJ2ArmLength * sin(toRadians(axisJ2)) + axisJ3CArmLength * sin(toRadians(axisJ3C))
], axisJ3CArmWidth / 2.01, %)
const extrude021 = extrude(-0.5, sketch021)
// Define Grabber Claw Constants
const grabberLength = 7
const sketch022 = startSketchOn(extrude021, 'START')
|> circle([0, 0], 0.10, %)
const extrude022 = extrude(-0.01, sketch022)
// Build Upper Claw Finger
const sketch023 = startSketchOn(extrude022, 'START')
|> startProfileAt([
1.75 + axisJ2ArmLength * cos(toRadians(axisJ2)) + axisJ3CArmLength * cos(toRadians(axisJ3C)),
8 + axisJ2ArmLength * sin(toRadians(axisJ2)) + axisJ3CArmLength * sin(toRadians(axisJ3C))
], %)
|> angledLine({
angle: axisJ3C + axisJ4 / 2,
length: grabberLength / 4
}, %)
|> arc({
angleStart: 150 + axisJ3C + axisJ4 / 2,
angleEnd: 30 + axisJ3C + axisJ4 / 2,
radius: grabberLength / 3
}, %)
|> angledLine({
angle: axisJ3C + axisJ4 / 2,
length: grabberLength / 6
}, %)
|> angledLine({
angle: axisJ3C + axisJ4 / 2 + 132,
length: grabberLength / 3.5
}, %)
|> angledLine({
angle: axisJ3C + axisJ4 / 2 + 160,
length: grabberLength / 3.5
}, %)
|> angledLine({
angle: axisJ3C + axisJ4 / 2 + 200,
length: grabberLength / 3
}, %)
|> lineTo([profileStartX(%), profileStartY(%)], %)
|> close(%)
const extrude023 = extrude(-1.5, sketch023)
// Build Lower Claw Finger
const sketch024 = startSketchOn(extrude022, 'START')
|> startProfileAt([
1.75 + axisJ2ArmLength * cos(toRadians(axisJ2)) + axisJ3CArmLength * cos(toRadians(axisJ3C)),
8 + axisJ2ArmLength * sin(toRadians(axisJ2)) + axisJ3CArmLength * sin(toRadians(axisJ3C))
], %)
|> angledLine({
angle: axisJ3C - (axisJ4 / 2),
length: grabberLength / 4
}, %)
|> arc({
angleStart: 210 + axisJ3C - (axisJ4 / 2),
angleEnd: 330 + axisJ3C - (axisJ4 / 2),
radius: grabberLength / 3
}, %)
|> angledLine({
angle: axisJ3C - (axisJ4 / 2),
length: grabberLength / 6
}, %)
|> angledLine({
angle: axisJ3C - (axisJ4 / 2) - 132,
length: grabberLength / 3.5
}, %)
|> angledLine({
angle: axisJ3C - (axisJ4 / 2) - 160,
length: grabberLength / 3.5
}, %)
|> angledLine({
angle: axisJ3C - (axisJ4 / 2) - 200,
length: grabberLength / 3
}, %)
|> lineTo([profileStartX(%), profileStartY(%)], %)
|> close(%)
const extrude024 = extrude(-1.5, sketch024)