kcl-samples → pipe-flange-assembly
pipe-flange-assembly
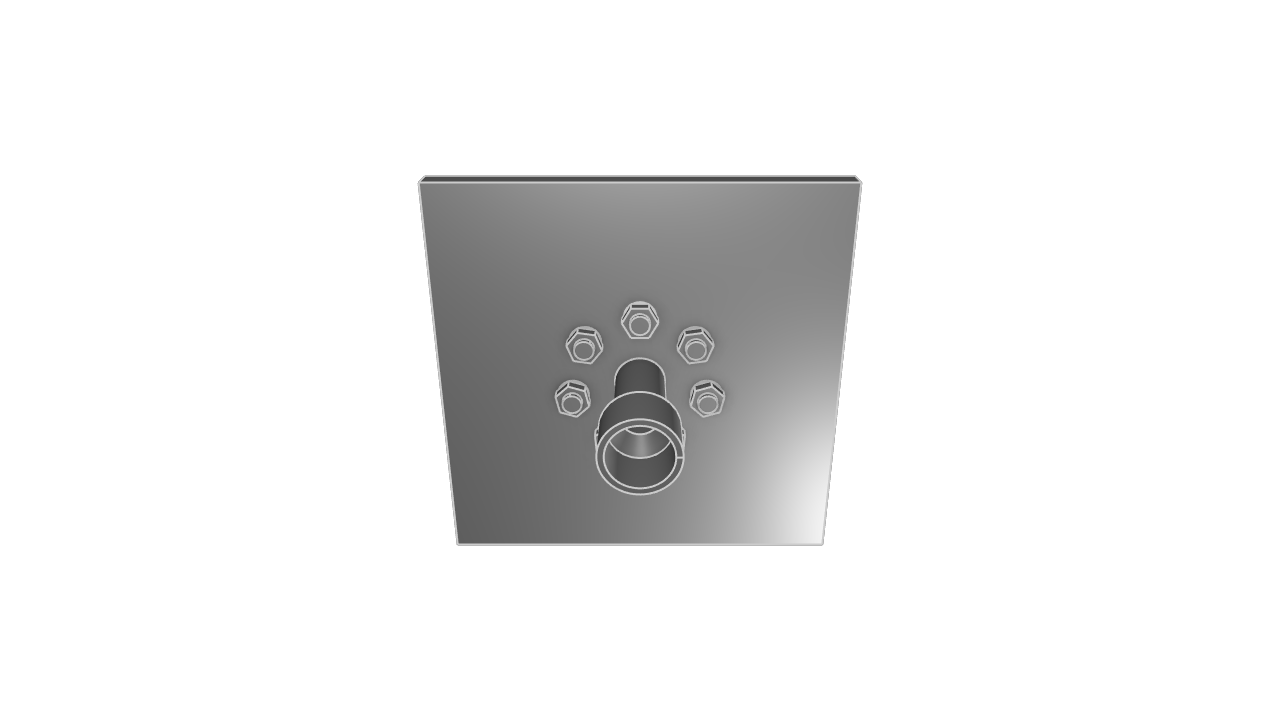
KCL
// Pipe and Flange Assembly
// A crucial component in various piping systems, designed to facilitate the connection, disconnection, and access to piping for inspection, cleaning, and modifications. This assembly combines pipes (long cylindrical conduits) with flanges (plate-like fittings) to create a secure yet detachable joint.
// Set units
@settings(defaultLengthUnit = in, kclVersion = 1.0)
// Import parameters
import * from "parameters.kcl"
// Import parts
import "9472k188-gasket.kcl" as gasket
import flange from "68095k348-flange.kcl"
import washer from "98017a257-washer.kcl"
import bolt from "91251a404-bolt.kcl"
import hexNut from "95479a127-hex-nut.kcl"
import pipe from "1120t74-pipe.kcl"
// Place flanges
flange()
flange()
|> rotate(axis = [0, 1, 0], angle = 180)
|> translate(x = 0, y = 0, z = flangeBackHeight * 2 + gasketThickness)
// Place gasket between the flanges
gasket
|> translate(x = 0, y = 0, z = -flangeBackHeight - gasketThickness)
// Place eight washers (four front, four back)
washer()
|> translate(x = mountingHolePlacementDiameter / 2, y = 0, z = flangeBaseThickness)
|> patternCircular3d(
%,
instances = 4,
axis = [0, 0, 1],
center = [0, 0, 0],
arcDegrees = 360,
rotateDuplicates = false,
)
|> patternLinear3d(
%,
instances = 2,
distance = -(flangeBaseThickness * 2 + flangeBackHeight * 2 + gasketThickness + washerThickness),
axis = [0, 0, 1],
)
// Place four bolts
bolt()
|> translate(x = mountingHolePlacementDiameter / 2, y = 0, z = flangeBaseThickness + washerThickness)
|> rotate(roll = 90, pitch = 0, yaw = 0)
|> patternCircular3d(
%,
instances = 4,
axis = [0, 0, 1],
center = [0, 0, 0],
arcDegrees = 360,
rotateDuplicates = false,
)
// Place four hex nuts
hexNut()
|> translate(x = mountingHolePlacementDiameter / 2, y = 0, z = -(flangeBackHeight * 2 + gasketThickness + flangeBaseThickness + washerThickness + hexNutThickness))
|> patternCircular3d(
%,
instances = 4,
axis = [0, 0, 1],
center = [0, 0, 0],
arcDegrees = 360,
rotateDuplicates = false,
)
// Place both pieces of pipe
pipe()
|> rotate(
%,
roll = -90,
pitch = 0,
yaw = 0,
)
|> translate(
%,
x = 0,
y = 0,
z = flangeBaseThickness + flangeFrontHeight - 0.5,
global = true,
)
pipe()
|> rotate(
%,
roll = 90,
pitch = 0,
yaw = 0,
)
|> translate(
%,
x = 0,
y = 0,
z = -(flangeBackHeight * 2 + gasketThickness + flangeBaseThickness + flangeFrontHeight - 0.5),
global = true,
)