kcl-samples → exhaust-manifold
exhaust-manifold
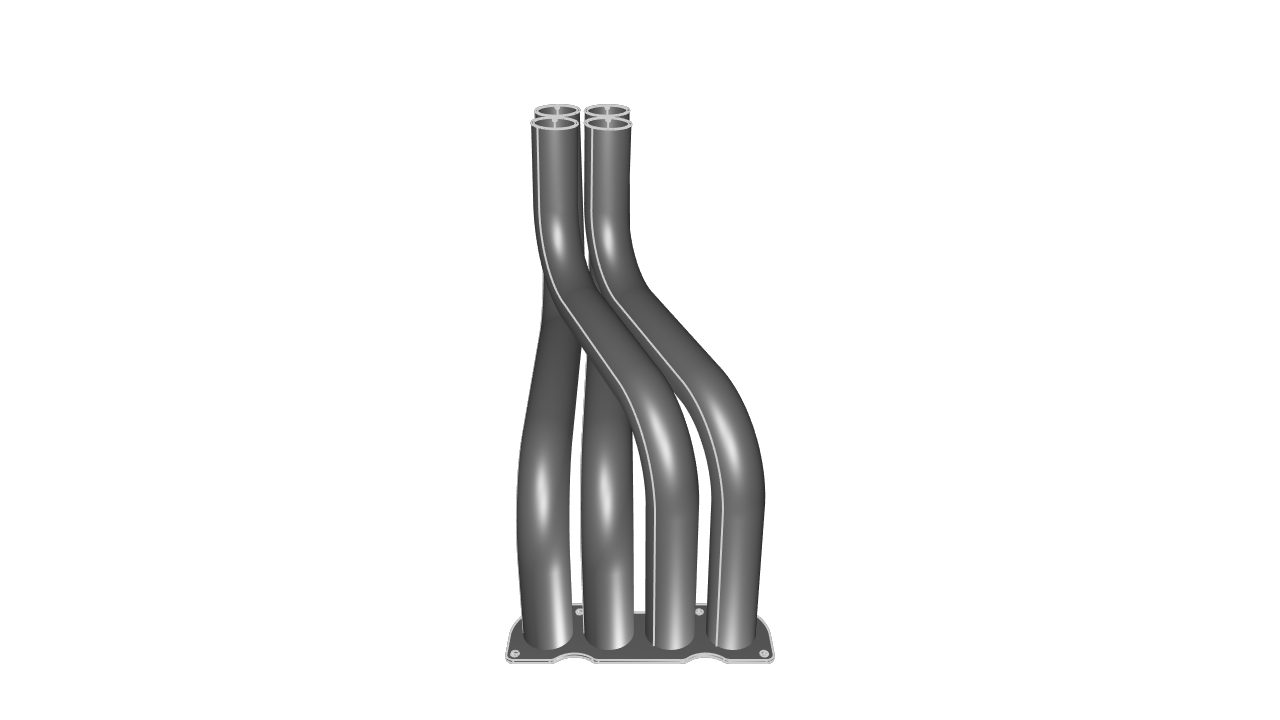
KCL
// Exhaust Manifold
// A welded exhaust header for an inline 4-cylinder engine
// Set units
@settings(defaultLengthUnit = in, kclVersion = 1.0)
// Define parameters
primaryTubeDiameter = 1.625
wallThickness = 0.080
plateHeight = 0.125
bendRadius = 3
// Create a function to draw each primary tube with specified lengths and angles
fn primaryTube(n, angle001, length001, length002, length003) {
// Create an index for the function
pos001 = n * 2
// Define a plane for each sweep path defined by an angle
sweepPlane = {
origin = [pos001, 0.0, 0],
xAxis = [sin(-angle001), cos(-angle001), 0.0],
yAxis = [0.0, 0.0, 1.0],
zAxis = [1.0, 0.0, 0.0]
}
// Draw a path for each sweep
sweepPath = startSketchOn(sweepPlane)
|> startProfile(at = [0, plateHeight])
|> line(end = [0, length001])
|> tangentialArc(angle = -80, radius = bendRadius, tag = $arc01)
|> angledLine(angle = tangentToEnd(arc01), length = length002)
|> tangentialArc(angle = 85, radius = bendRadius, tag = $arc02)
|> angledLine(angle = tangentToEnd(arc02), length = length003)
// Create the cross section of each tube and sweep them
sweepProfile = startSketchOn(XY)
|> circle(center = [pos001, 0], radius = primaryTubeDiameter / 2)
|> subtract2d(tool = circle(center = [pos001, 0], radius = primaryTubeDiameter / 2 - wallThickness))
|> sweep(path = sweepPath)
return { }
}
// Draw a primary tube for each cylinder with specified lengths and angles
primaryTube(
n = 0,
angle001 = 0,
length001 = 3,
length002 = 6,
length003 = 5,
)
primaryTube(
n = 1,
angle001 = 1,
length001 = 3,
length002 = 6,
length003 = 5,
)
primaryTube(
n = 2,
angle001 = 24.3,
length001 = 5,
length002 = 5,
length003 = 3,
)
primaryTube(
n = 3,
angle001 = 25.2,
length001 = 5,
length002 = 5,
length003 = 3,
)
// Create the mounting flange for the header
flangeSketch = startSketchOn(XY)
|> startProfile(at = [3 + 1.3, -1.25])
|> xLine(length = -2.6, tag = $seg01)
|> tangentialArc(radius = .3, angle = -40)
|> tangentialArc(radius = .9, angle = 80)
|> tangentialArc(radius = .3, angle = -40)
|> xLine(length = -1.4, tag = $seg03)
|> yLine(length = segLen(seg01), tag = $seg04)
|> xLine(length = 3.1, tag = $seg05)
|> tangentialArc(radius = .3, angle = -40)
|> tangentialArc(radius = 1.5, angle = 80)
|> tangentialArc(radius = .3, angle = -40)
|> xLine(length = segLen(seg05), tag = $seg07)
|> yLine(endAbsolute = profileStartY(%), tag = $seg08)
|> xLine(length = -segLen(seg03), tag = $seg09)
|> tangentialArc(radius = .3, angle = -40)
|> tangentialArc(radius = .9, angle = 80)
|> tangentialArc(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
// Create openings in the flange to accommodate each tube
|> subtract2d(tool = circle(center = [0, 0], radius = primaryTubeDiameter / 2 - wallThickness))
|> subtract2d(tool = circle(center = [2, 0], radius = primaryTubeDiameter / 2 - wallThickness))
|> subtract2d(tool = circle(center = [4, 0], radius = primaryTubeDiameter / 2 - wallThickness))
|> subtract2d(tool = circle(center = [6, 0], radius = primaryTubeDiameter / 2 - wallThickness))
// Add mounting holes to the flange
|> subtract2d(tool = circle(
center = [
-primaryTubeDiameter * .6,
-primaryTubeDiameter * .6
],
radius = 0.25 / 2,
))
|> subtract2d(tool = circle(
center = [
primaryTubeDiameter * .6,
primaryTubeDiameter * .6
],
radius = 0.25 / 2,
))
|> subtract2d(tool = circle(
center = [
3 * 2 - (primaryTubeDiameter * .6),
primaryTubeDiameter * .6
],
radius = 0.25 / 2,
))
|> subtract2d(tool = circle(
center = [
3 * 2 + primaryTubeDiameter * .6,
-primaryTubeDiameter * .6
],
radius = 0.25 / 2,
))
// Extrude the flange and fillet the edges
|> extrude(length = plateHeight)
|> fillet(
radius = 1.5,
tags = [
getNextAdjacentEdge(seg04),
getNextAdjacentEdge(seg07)
],
)
|> fillet(
radius = .25,
tags = [
getNextAdjacentEdge(seg03),
getNextAdjacentEdge(seg08)
],
)