kcl-samples → gear →
gear
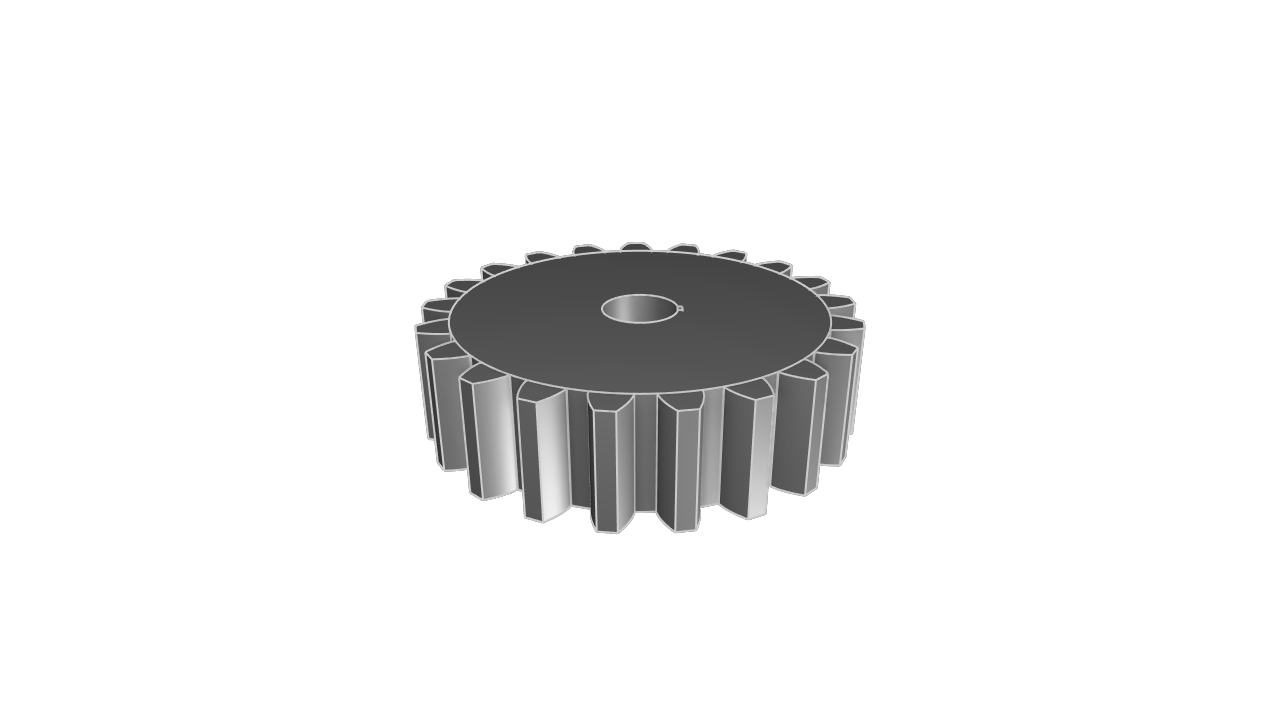
KCL
// Spur Gear
// A rotating machine part having cut teeth or, in the case of a cogwheel, inserted teeth (called cogs), which mesh with another toothed part to transmit torque. Geared devices can change the speed, torque, and direction of a power source. The two elements that define a gear are its circular shape and the teeth that are integrated into its outer edge, which are designed to fit into the teeth of another gear.
// Define constants
nTeeth = 21
module = 0.5
pitchDiameter = module * nTeeth
pressureAngle = 20
addendum = module
deddendum = 1.25 * module
baseDiameter = pitchDiameter * cos(toRadians(pressureAngle))
tipDiameter = pitchDiameter + 2 * module
gearHeight = 3
// Interpolate points along the involute curve
cmo = int(101)
rs = map([0..cmo], (i) => {
return baseDiameter / 2 + i / cmo * (tipDiameter - baseDiameter) / 2
})
// Calculate operating pressure angle
angles = map(rs, (r) => {
return toDegrees( acos(baseDiameter / 2 / r))
})
// Calculate the involute function
invas = map(angles, (a) => {
return tan(toRadians(a)) - toRadians(a)
})
// Map the involute curve
xs = map([0..cmo], (i) => {
return rs[i] * cos(invas[i])
})
ys = map([0..cmo], (i) => {
return rs[i] * sin(invas[i])
})
// Extrude the gear body
body = startSketchOn('XY')
|> circle({
center = [0, 0],
radius = baseDiameter / 2
}, %)
|> extrude(gearHeight, %)
toothAngle = 360 / nTeeth / 1.5
// Plot the involute curve
fn leftInvolute(i, sg) {
j = int(100 - i) // iterate backwards
return lineTo([xs[j], ys[j]], sg)
}
fn rightInvolute(i, sg) {
x = rs[i] * cos(toRadians(-toothAngle + toDegrees(atan(ys[i] / xs[i]))))
y = -rs[i] * sin(toRadians(-toothAngle + toDegrees(atan(ys[i] / xs[i]))))
return lineTo([x, y], sg)
}
// Draw gear teeth
start = startSketchOn('XY')
|> startProfileAt([xs[101], ys[101]], %)
teeth = reduce([0..100], start, leftInvolute)
|> arc({
angleStart = 0,
angleEnd = toothAngle,
radius = baseDiameter / 2
}, %)
|> reduce([1..101], %, rightInvolute)
|> close(%)
|> extrude(gearHeight, %)
|> patternCircular3d({
axis = [0, 0, 1],
center = [0, 0, 0],
instances = nTeeth,
arcDegrees = 360,
rotateDuplicates = true
}, %)
// Define the constants of the keyway and the bore hole
keywayWidth = 0.250
keywayDepth = keywayWidth / 2
holeDiam = 2
holeRadius = 1
startAngle = asin(keywayWidth / 2 / holeRadius)
// Sketch the keyway and center hole and extrude
keyWay = startSketchOn(body, 'END')
|> startProfileAt([
holeRadius * cos(startAngle),
holeRadius * sin(startAngle)
], %)
|> xLine(keywayDepth, %)
|> yLine(-keywayWidth, %)
|> xLine(-keywayDepth, %)
|> arc({
angleEnd = 180,
angleStart = -1 * 180 / pi() * startAngle + 360,
radius = holeRadius
}, %)
|> arc({
angleEnd = 180 / pi() * startAngle,
angleStart = 180,
radius = holeRadius
}, %)
|> close(%)
|> extrude(-gearHeight, %)