kcl-samples → wheel-rotor →
wheel-rotor
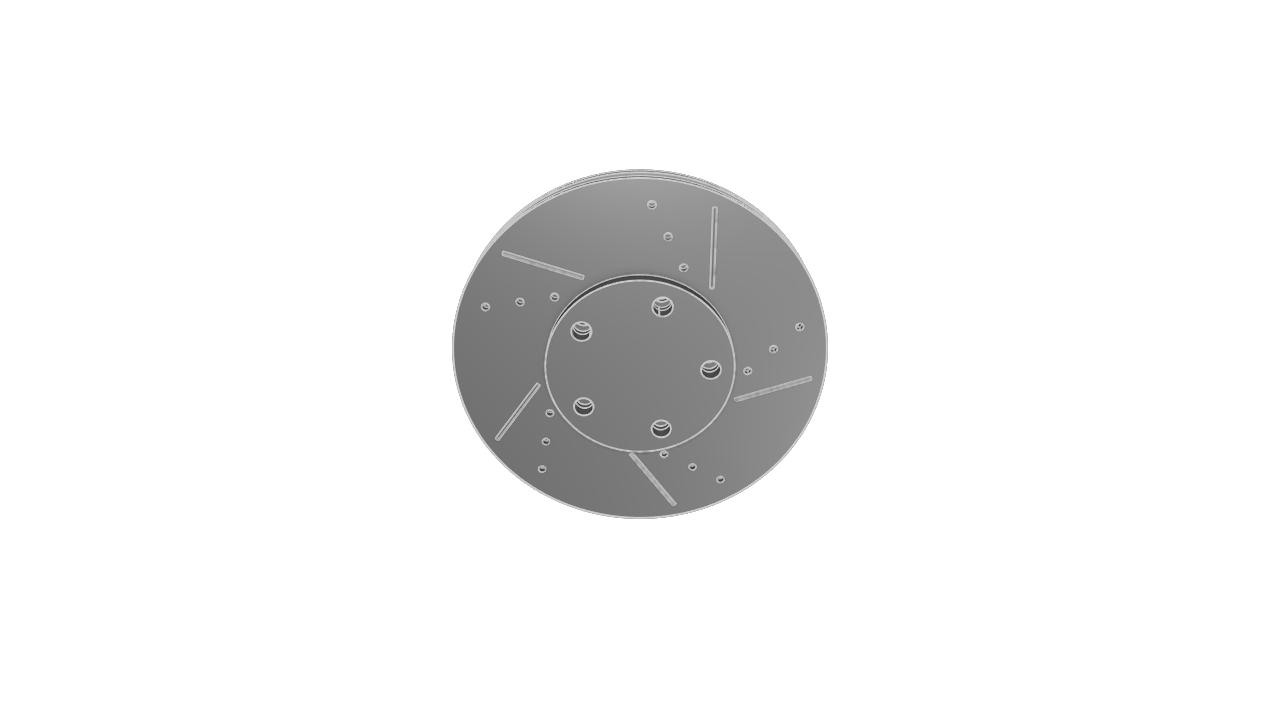
KCL
// Wheel rotor
// A component of a disc brake system. It provides a surface for brake pads to press against, generating the friction needed to slow or stop the vehicle.
// Define constants
// Base units for this model are in inches
const rotorDiameter = 12
const rotorInnerDiameter = 6
const rotorSinglePlateThickness = 0.25
const rotorInnerDiameterThickness = 0.5
const lugHolePatternDia = 3
const lugSpacing = 114.3 * mm()
const rotorTotalThickness = 1
const spacerPatternDiameter = 11
const spacerDiameter = 0.25
const spacerLength = rotorTotalThickness - (2 * rotorSinglePlateThickness)
const spacerCount = 16
const wheelDiameter = 19
const lugCount = 5
const yAxisOffset = 0.5
const drillAndSlotCount = 5
const rotorPlane = {
plane: {
origin: { x: 0, y: yAxisOffset, z: 0 },
xAxis: { x: -1, y: 0, z: 0 },
yAxis: { x: 0, y: 0, z: 1 },
zAxis: { x: 0, y: 1, z: 0 }
}
}
fn lugPattern = (plane) => {
const lugHolePattern = circle([-lugSpacing / 2, 0], 0.315, plane)
|> patternCircular2d({
arcDegrees: 360,
center: [0, 0],
repetitions: lugCount - 1,
rotateDuplicates: true
}, %)
return lugHolePattern
}
const rotorSketch = startSketchOn(rotorPlane)
|> circle([0, 0], rotorDiameter / 2, %)
|> hole(lugPattern(%), %)
const rotor = extrude(rotorSinglePlateThickness, rotorSketch)
const rotorBumpSketch = startSketchOn(rotorPlane)
|> circle([0, 0], rotorInnerDiameter / 2, %)
|> hole(lugPattern(%), %)
const rotorBump = extrude(-rotorInnerDiameterThickness, rotorBumpSketch)
const rotorSecondaryPlatePlane = {
plane: {
origin: {
x: 0,
y: yAxisOffset + rotorTotalThickness * 0.75,
z: 0
},
xAxis: { x: -1, y: 0, z: 0 },
yAxis: { x: 0, y: 0, z: 1 },
zAxis: { x: 0, y: 1, z: 0 }
}
}
const secondaryRotorSketch = startSketchOn(rotorSecondaryPlatePlane)
|> circle([0, 0], rotorDiameter / 2, %)
|> hole(lugPattern(%), %)
const secondRotor = extrude(rotorSinglePlateThickness, secondaryRotorSketch)
const spacerSketch = startSketchOn(rotorSecondaryPlatePlane)
|> circle([spacerPatternDiameter / 2, 0], spacerDiameter, %)
|> patternCircular2d({
arcDegrees: 360,
center: [0, 0],
repetitions: spacerCount,
rotateDuplicates: true
}, %)
const spacers = extrude(-spacerLength, spacerSketch)
const rotorSlottedSketch = startSketchOn(rotor, 'START')
|> startProfileAt([2.17, 2.56], %)
|> xLine(0.12, %)
|> yLine(2.56, %)
|> xLine(-0.12, %)
|> lineTo([profileStartX(%), profileStartY(%)], %)
|> close(%)
|> patternCircular2d({
center: [0, 0],
repetitions: drillAndSlotCount - 1,
arcDegrees: 360,
rotateDuplicates: true
}, %)
const rotorSlotted = extrude(-rotorSinglePlateThickness / 2, rotorSlottedSketch)
const rotorDrilledSketch = startSketchOn(rotor, 'START')
|> circle([1.34, 3.23], 0.12, %)
|> patternLinear2d({
axis: [-0.02, 0.04],
repetitions: 2,
distance: 1.1
}, %)
|> patternCircular2d({
center: [0, 0],
repetitions: drillAndSlotCount - 1,
arcDegrees: 360,
rotateDuplicates: true
}, %)
const rotorDrilled = extrude(-rotorSinglePlateThickness, rotorDrilledSketch)
const secondRotorSlottedSketch = startSketchOn(secondRotor, 'END')
|> startProfileAt([-2.17, 2.56], %)
|> xLine(-0.12, %)
|> yLine(2.56, %)
|> xLine(0.12, %)
|> lineTo([profileStartX(%), profileStartY(%)], %)
|> close(%)
|> patternCircular2d({
center: [0, 0],
repetitions: drillAndSlotCount - 1,
arcDegrees: 360,
rotateDuplicates: true
}, %)
const secondRotorSlotted = extrude(-rotorSinglePlateThickness / 2, secondRotorSlottedSketch)
const secondRotorDrilledSketch = startSketchOn(secondRotor, 'START')
|> circle([1.34, 3.23], 0.12, %)
|> patternLinear2d({
axis: [-0.02, 0.04],
repetitions: 2,
distance: 1.1
}, %)
|> patternCircular2d({
center: [0, 0],
repetitions: drillAndSlotCount - 1,
arcDegrees: 360,
rotateDuplicates: true
}, %)
const secondRotorDrilled = extrude(-rotorSinglePlateThickness, secondRotorDrilledSketch)